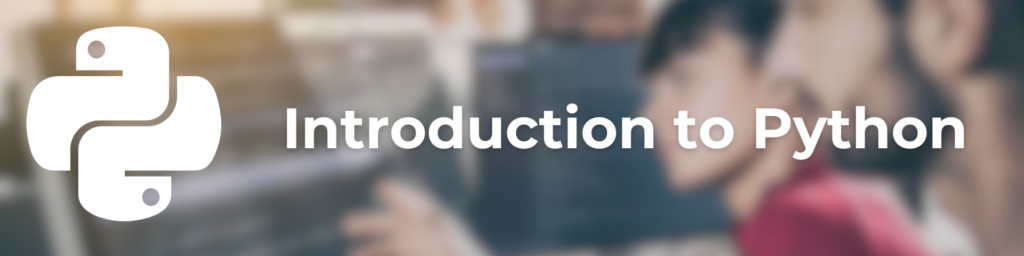
Description
This 3 day course is a comprehensive three-day program designed to teach Python programming. It covers a range of topics including Python basics, data types, collections, functions, object-oriented programming, modules, exception handling, file management, and database access. The course is structured into seven modules, each focusing on a specific aspect of Python with multiple hands-on labs in each module.
Audience
This course is well-suited for those looking to enhance their programming skills and understanding of Python for application development and data management tasks.
Prerequisites
-
There are no prerequisites required for this course.
Objectives
Six Core Concepts
-
- Efficiently develop Python applications by utilizing built-in functions and data structures.
- Apply object-oriented principles to structure your code, incorporating modules and classes.
- Demonstrate proficiency in working with functions and collections through practical exercises.
- Utilize external libraries to extend functionalities and manage various data formats effectively.
- Acquire hands-on experience in file and data handling within the Python environment.
- Develop comfort and competence in dealing with Exceptions through practical exception handling exercises.
Modules
Module 1: Python Introduction
- Background, Uses, and Characteristics
- Syntax and Writing Basic Code
- Data Types and Variables
- Operators
Module 2: Control Structures
-
- Conditional Statements
- if
- else
- elif
- Loops
- for
- while
- Loop Control Statements
- break
- continue
- pass
- Nested Control Structures
- Conditional Statements
Module 3: Collections
-
- Lists
- Tuples
- Dictionaries
- Sets
- Membership Testing
- Iterate Collections
- List Comprehensions
Module 4: Functions
-
- Create Functions Using def Keyword
- Arguments
- Positional
- Keyword
- Default
- Variable-Length
- Return Statements
- Scope
- Anonymous/Lambda Functions
- Higher Order Functions
Module 5: Object-Oriented Programming with Python
-
- Classes
- Objects
- Inheritance
- Encapsulation
- Polymorphism
- Creating Classes and Instantiating Objects
- Instance Methods
- Class Variables and Instance Variables
- Inheritance and Subclassing
Module 6: Python Modules
-
- Concept and Purpose of Modules in Python
- Creating and Using Modules
- import Statement
- Built-In Modules
- Creating Custom Modules
Module 7: Exceptions
-
- Exceptions vs. Syntax Errors
- Exception Handling
- try Block
- except Block
- else Clause
- finally Block
- Multiple Exception Handling
- Raising Exceptions
- Custom Exception Classes